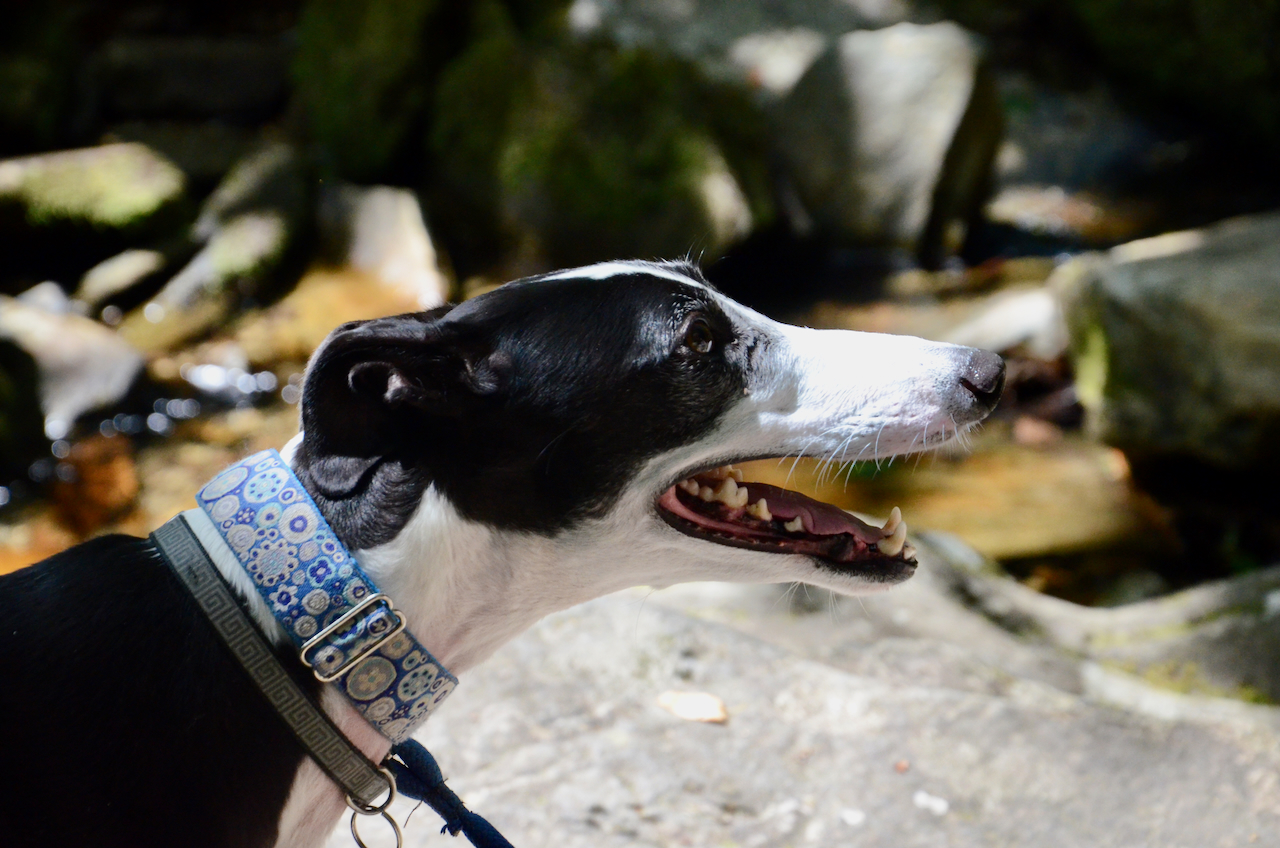
Thoughts on Professional Development
Ongoing professional development is a fact of life for people who want a long-term career in technology. That means finding ways to learn new skills above and beyond what you’re paid to do. We all need to strike a balance between having a life outside of work, and making sure we’re learning new work-related skills. Your professional development should not take over your personal life, but if you only learn on the job you will limit your options over time and be bound to the generosity of your employer. ...
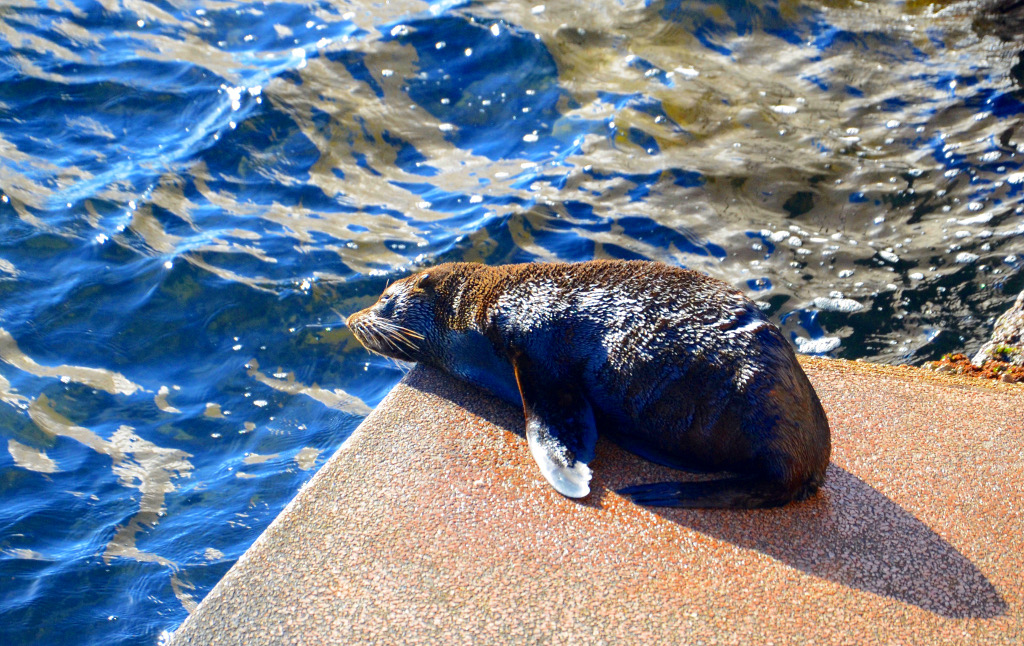
Welcome to Hugo
After several months of effort (not daily – I took my sweet time) I have converted this blog from WordPress to Hugo. That conversion has brought a modernized look and feel, and a lot of updates of old content. I moved away from plugins and short codes that WordPress used to theme modifications to support the short codes of Hugo. It’s forced me to learn a new template engine, platform, and web site paradigm. It’s been a bit of a journey to get here, so I figured I’d explain why I bothered and the work that went into the change. ...
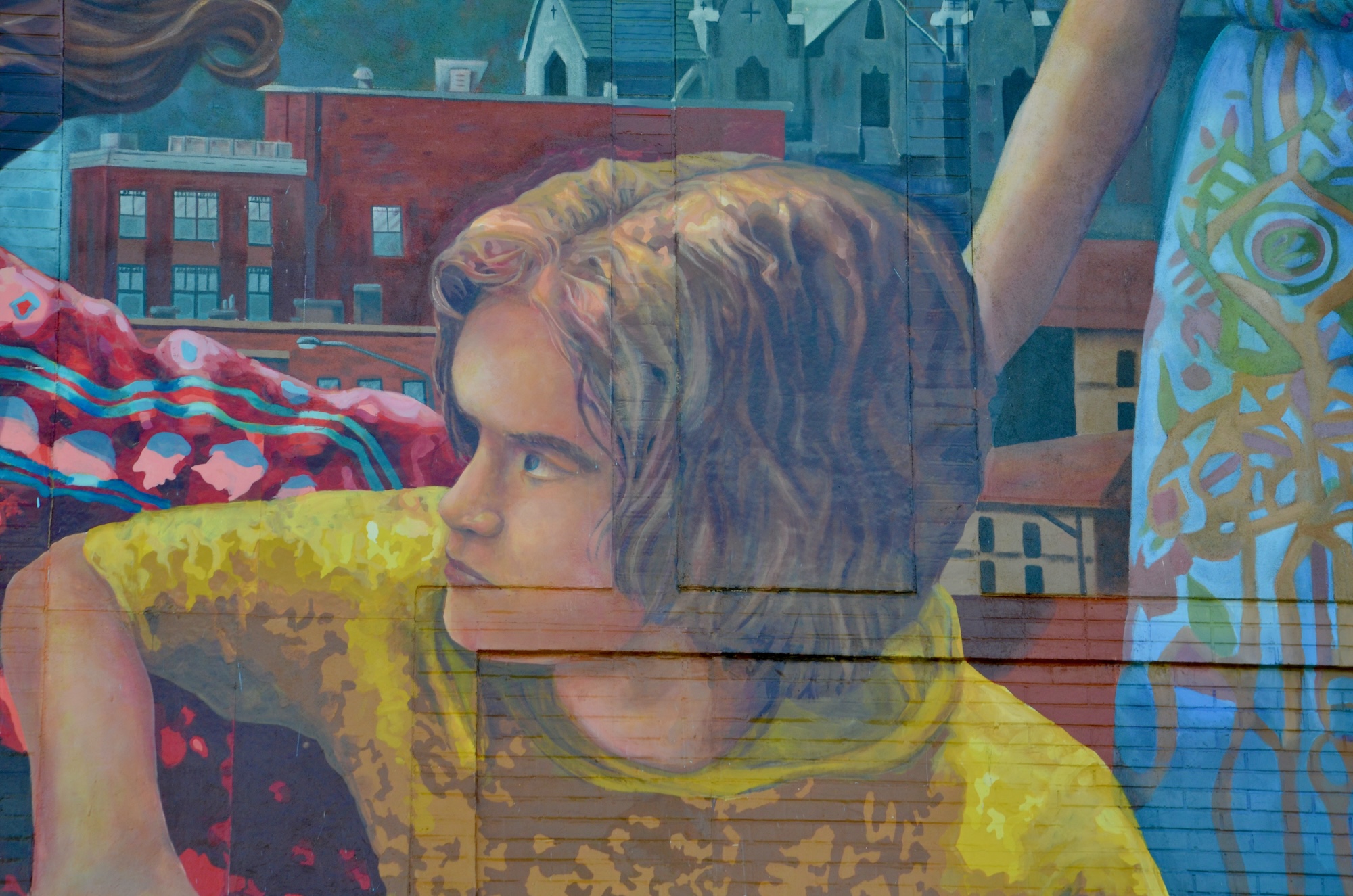
My Self Documenting Code Doesn't Need to be in Git: and Other Lies Salesforce Developers Tell Themselves
One of the things that consistently surprises me as a developer in the Salesforce eco system are the lies developers are comfortable telling themselves. Some lies (like that their code is self-documenting) are common developer myths. Others (like not needing git) are Salesforce community specific. So, as a public service to the community, I provide here a list of common lies and a sentence or two about why you should stop telling them. ...
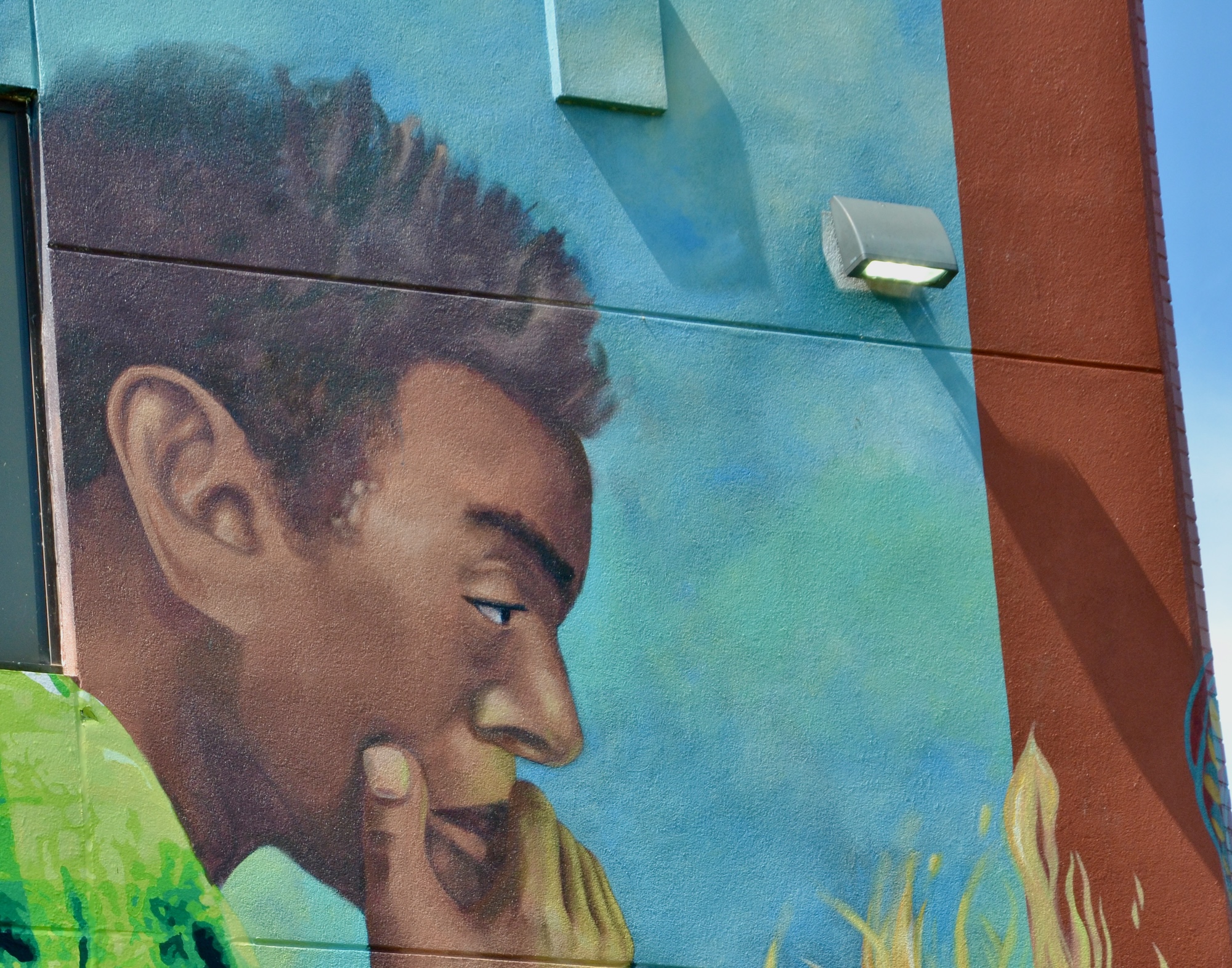
Writing for Developers and Consultants: Guides and Resources
Last year I wrote several pieces on writing for Developer and Consultants (the writing category will bring them up). That series became a talk on broader communications skills that I gave at Dreamforce 2024, and other settings – including twice this month. Part of that talk includes a series of books and similar resources that weren’t part of the original series. I figured it was time to add them in here. ...
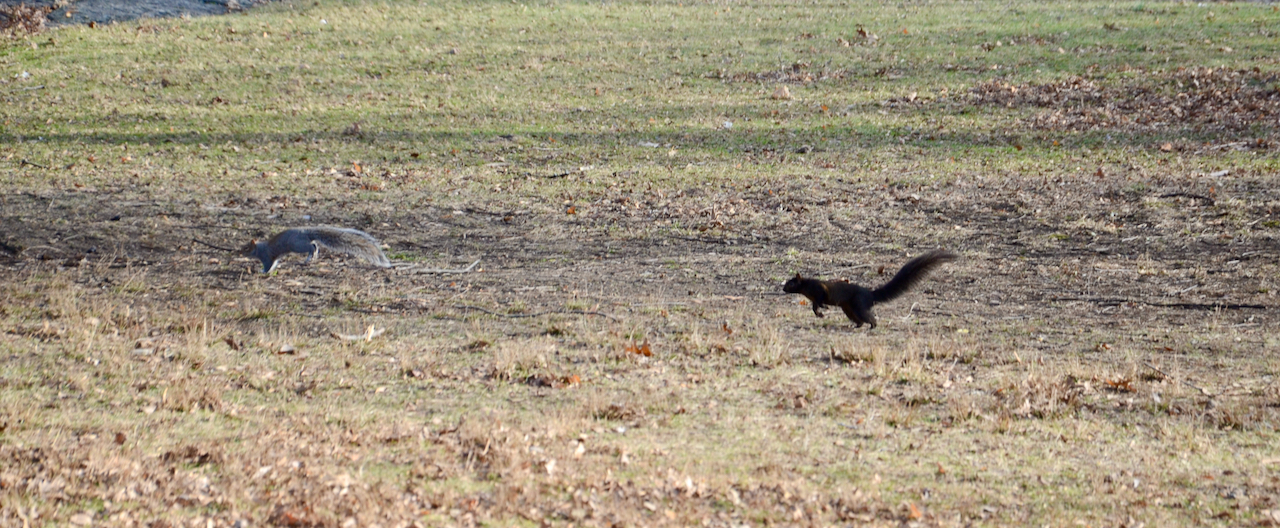
Salesforce Id Iteration Attacks
Please stop using Salesforce Ids in URLs or other user accessible parts of your solution. Why not use Salesforce Ids on public web sites? I run into this question from time to time and figured some more public commentary would be useful. The super short version: they are not a security or access control mechanism. During a recent Salesforce Open Source Commons sprint a question came up about why it’s important to use a random value, instead of a Salesforce Id, in public facing use cases. We were talking about the Unsubscribe Link package, and it makes a good use case for discussion. ...
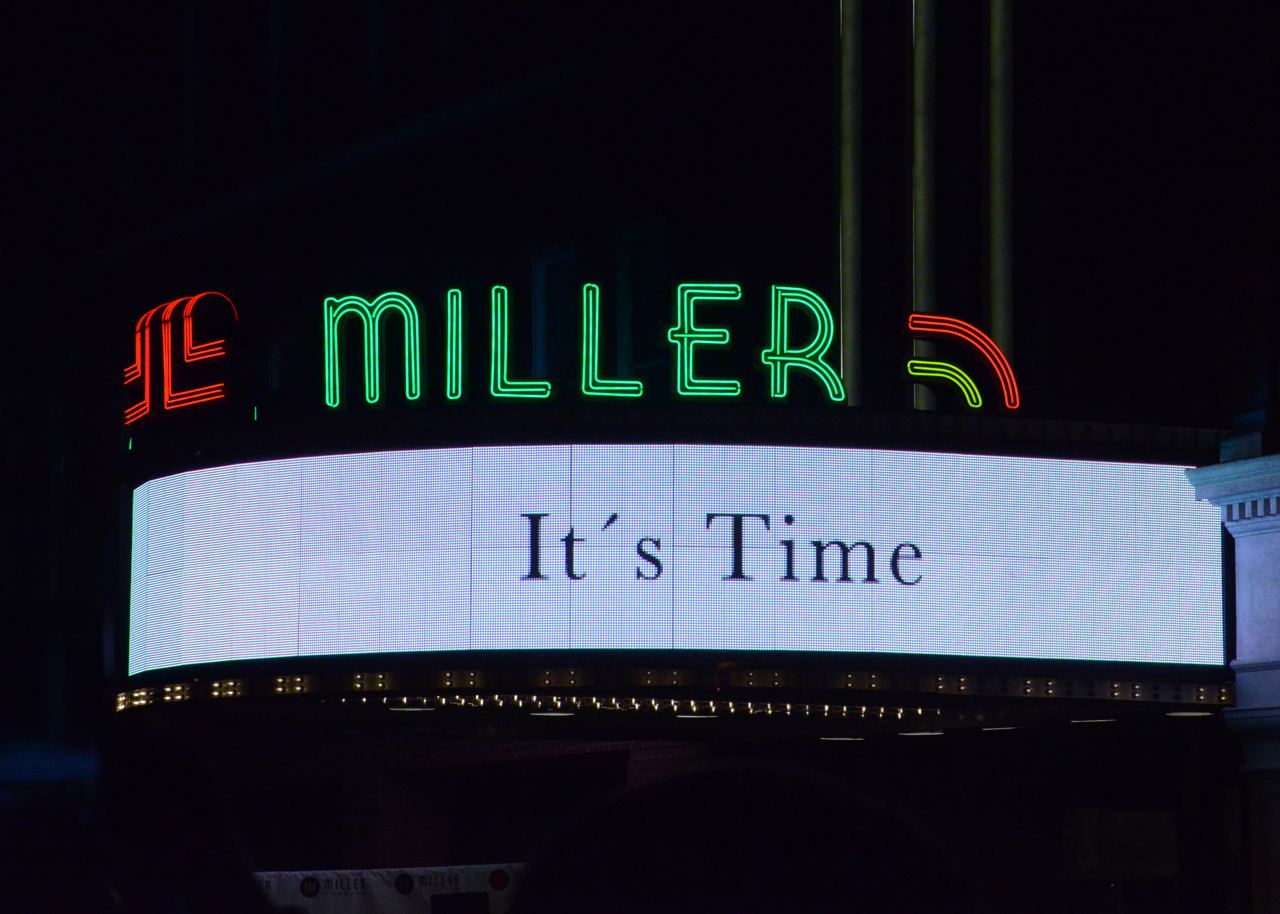
Experiments with AI Coding
I recently spent some time generating some simple programs with AI coding tools. As a technical architect and senior developer, I am watching the emergence of AI coding tools carefully. I know that the robots are coming for the coding parts of my job. Watching this generation of robots is important to long-term understanding of the field. To be clear I have been experimenting with Github Copilot and Salesforce’s Agentforce for Developers since they were released. Most of my earlier experiments were failures. The tools wrote trivial code terribly and simply couldn’t understand more complex requests. They made me slower, not faster, so I put them aside for a time. But they are evolving fast and were worth another look. ...
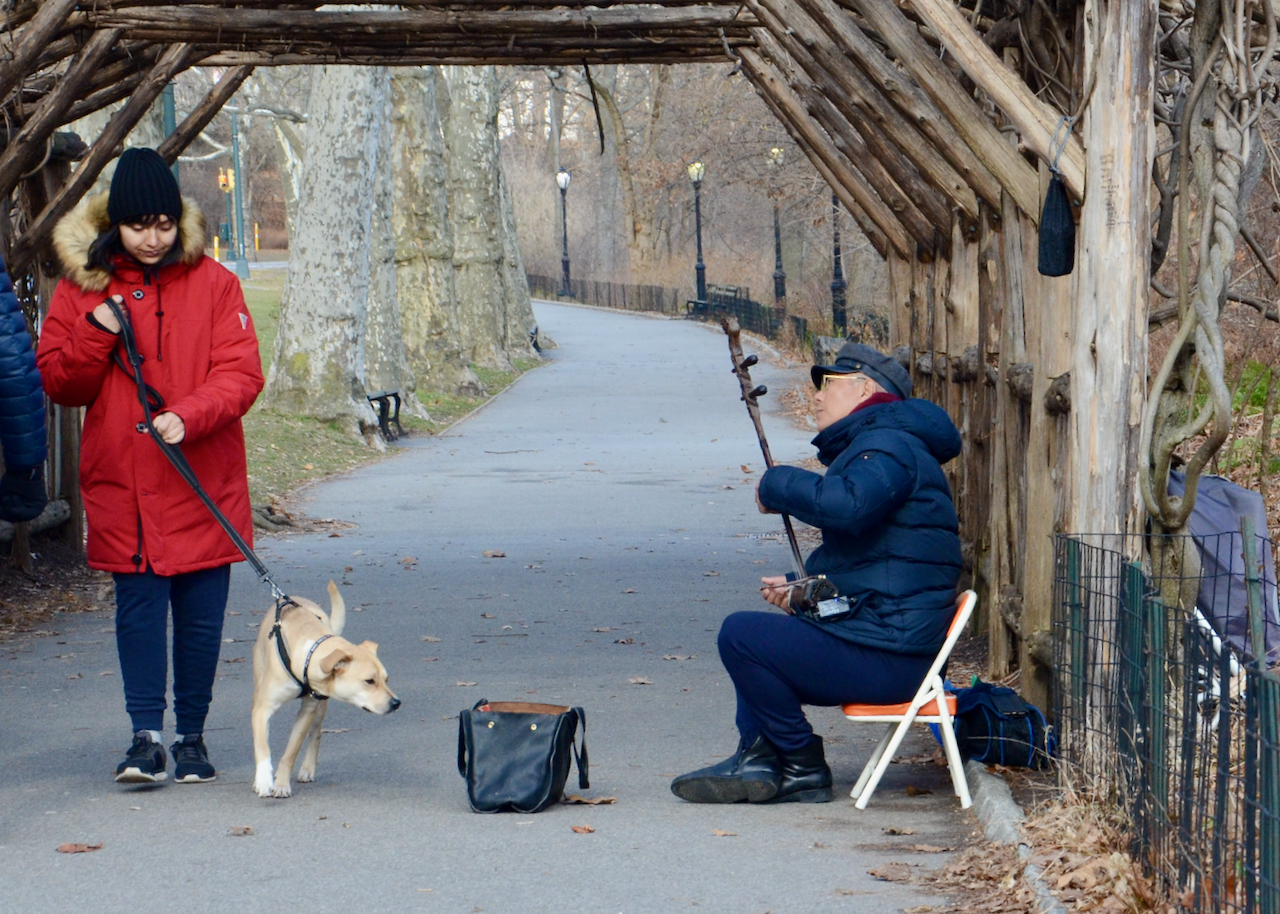
Reset Security Token for Salesforce Integration User
In the Spring ’23 release Salesforce added the Integration User License. They provide 1 or more free licenses to all Enterprise, Unlimited, Performance, and Developer Edition orgs. They can be a little tricky to setup, but are incredibly useful for integrations since they give you a free way to connect 3rd party applications where “best practice” had previously required buying a full license for each integration point. But because they have no user interface access there is no clear path to reset the security token (in fact Salesforce says you cannot do it, although it’s listed as on Roadmap). There is a way to get this done, it just requires that you have Login As permission. ...
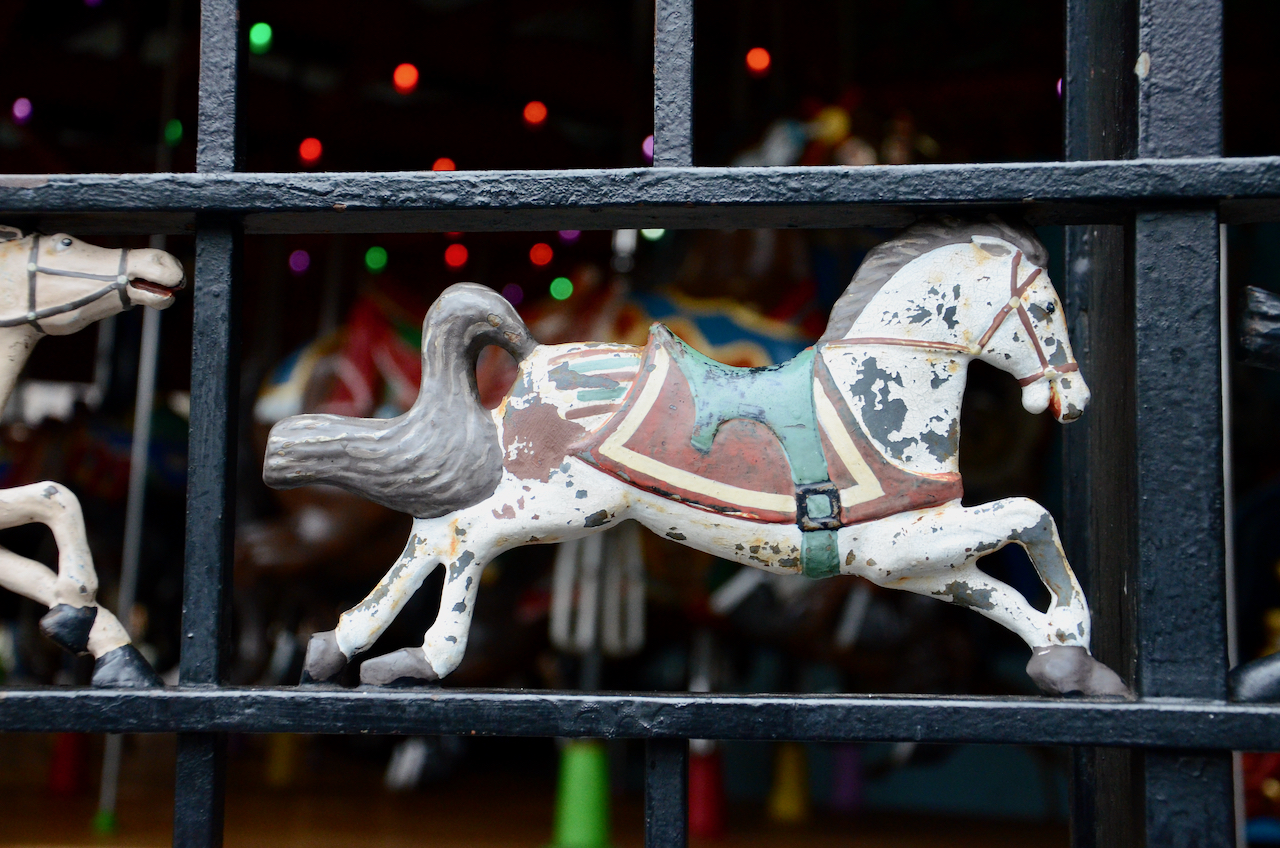
Architectures for Constituent Portals Part 2
In part 1 I talked about the kinds of architectures you should considering when planning a Constituent portal. In part 2 I’ll give you some guidance about how to pick the right architecture for your project. Reminder of the Options Part 1 provides a detailed review of the directions that data can flow, and the types of architectures you can use to support your solution: Data can be outbound to constituents, provided by individual constituents, or exchanged among constituents. There are all-in-one solutions, two-system solutions, and solutions with an API or data proxy layer. Three patterns, three potential solutions: it’s temping to think they align 1:1 – but then a whole second article would have been a waste of time. But it is important to keep them in your mind as you determine the best-fit for your organization and use case(s). ...
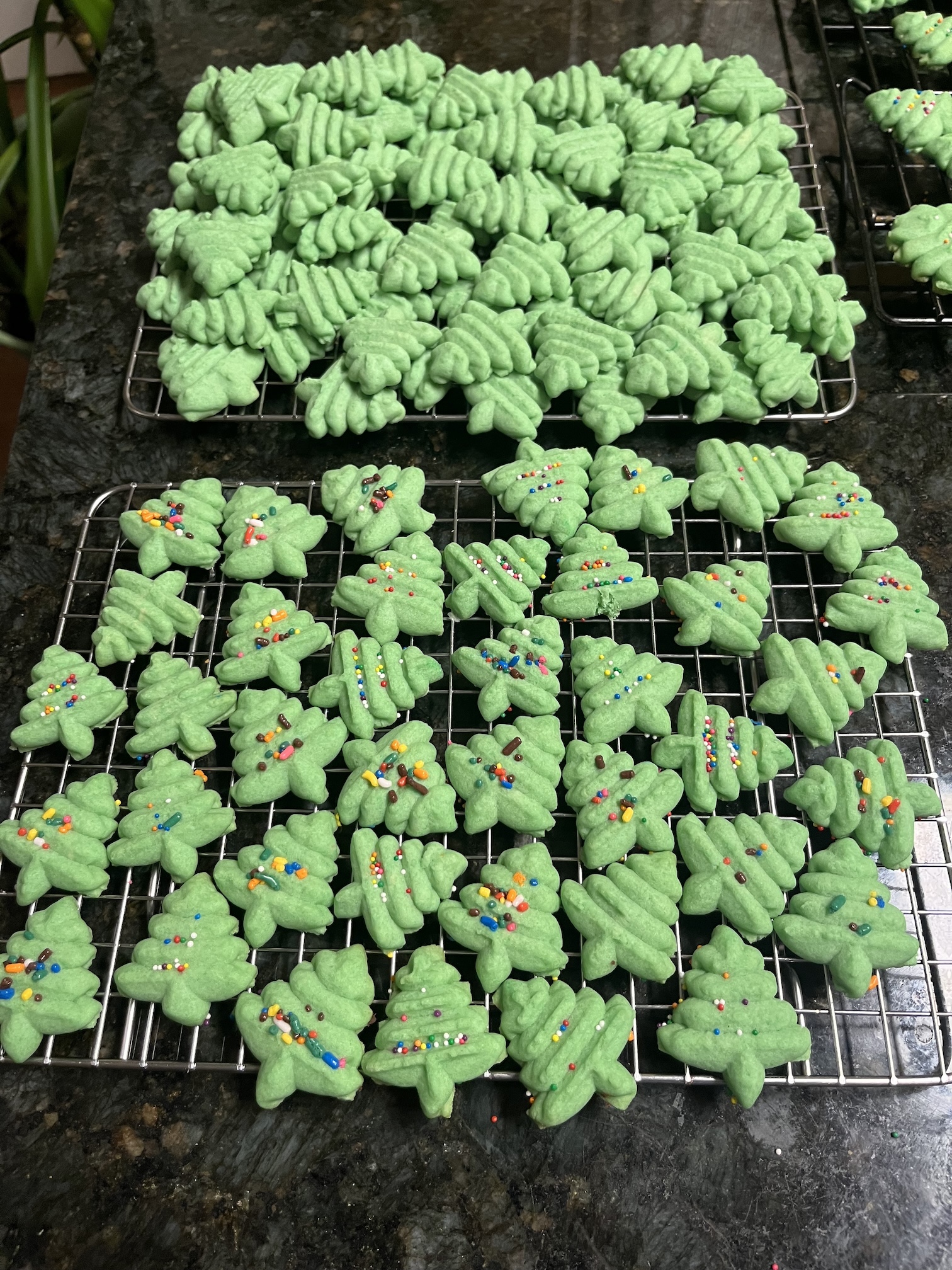
Mom's Christmas Cookies
My mom passed away just over a year ago. We went through Christmas without her last year for the first time, but there is still a different feel this year. One of the ways both my sister and I remember mom is through her baking. There were several classic cookies, and similar treats, she made every year. Neither of us makes the whole collection, but we both have settled into a couple favorites. ...
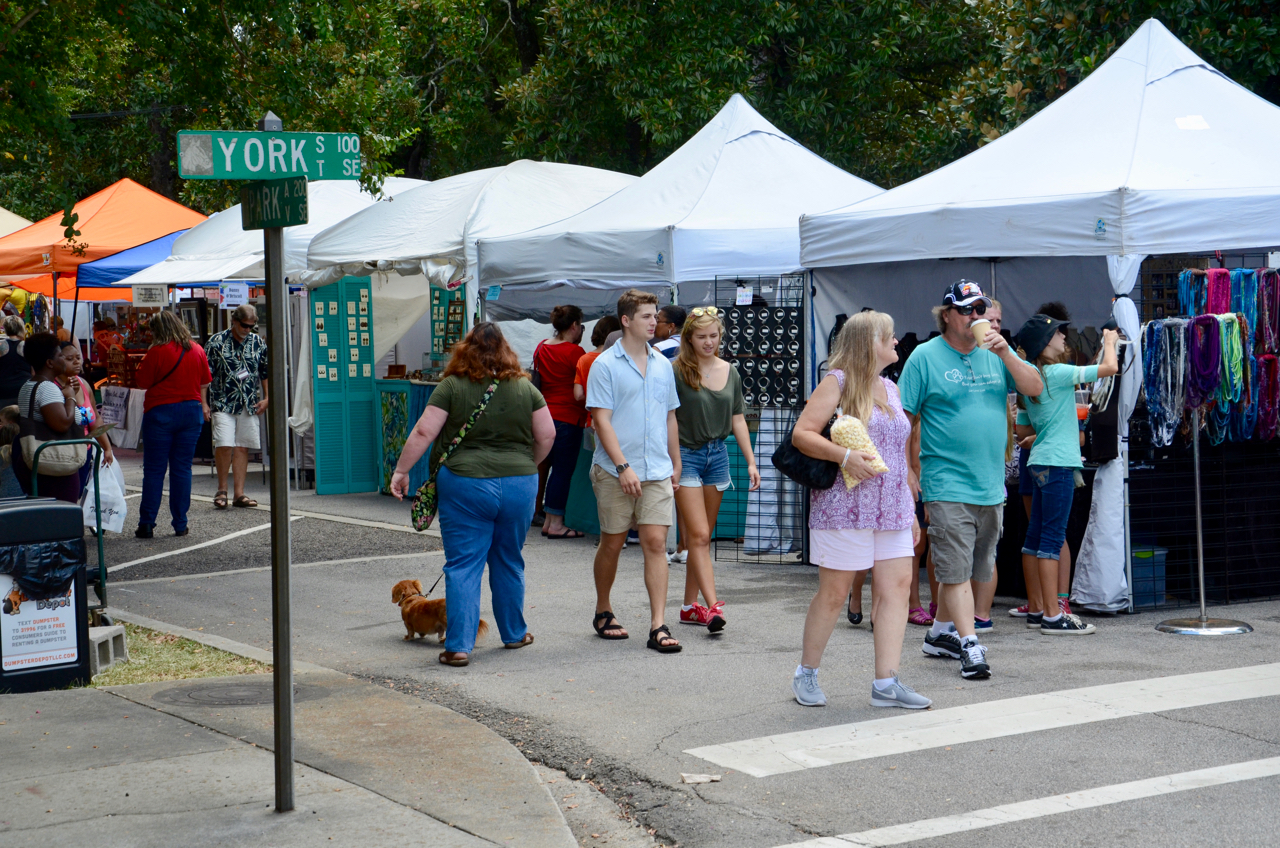
Architectures for Constituent Portals
One of the common needs, or at least desires, for CRM users is to link their CRM to some kind of constituent portal. There are several ways you can architect a good data pattern for your constituent portal. The trick is picking the right one for your organization. This is the first in a series on what your choices are, and now to select the right one. The architecture you select will drive the implementation cost, maintenance costs, and scalability. ...